Cheatsheet
A reference for often-used lines of code and commands.
Table of Contents:
CSS
For a more detailed explanation check out my positioning tutorial.
Absolute Positioning:
.parent {
position: relative;
}
.child {
position: absolute;
top: 10px;
left: 10px;
}
Flexbox:
.container {
display: flex;
justify-content: center; // horizontal
align-items: center; // vertical
}
Grid:
.container {
display: grid;
grid-gap: 10px;
grid-template:
"header header"
"sidebar main"
"footer footer"
/ 100px 1fr;
}
header { grid-area: header; }
aside { grid-area: sidebar; }
main { grid-area: main; }
footer { grid-area: footer; }
Animation:
@keyframes floatyAnimation {
0% {
transform: translateY(0px);
}
50% {
transform: translateY(-4px);
}
100% {
transform: translateY(0px);
}
}
div {
animation: floatyAnimation 2s ease-in-out infinite;
@media (prefers-reduced-motion) {
animation: none;
}
}
JavaScript
Waiting for DOM:
document.addEventListener("DOMContentLoaded", () => {
// code here...
});
For-Each over Elements:
const myElements = document.querySelectorAll(".element");
[...myElements].forEach((el) => {
console.log(el);
});
ECMAScript:
import "./myStyles.scss";
import "./myFolder/myFile";
import { doSomething } from "./myFolder/myFile";
export function doSomething() {
...
}
fetch
:
fetch("/example.json")
.then(response => {
// handle the response
if (res.ok) {
console.log("ok! (200)");
}
response.json();
})
.then(response => {
console.log(response); // e.g. response.json();
})
.catch(error => {
// handle the error. note: 404 errors do not land here.
console.log(error);
});
JSON -> JS Object: jsonData.json()
JS Object -> JSON: JSON.stringify(obj)
SCSS
$font: Helvetica, Arial;
$textColor: black;
$linkColor: #333333;
body {
font: $font;
color: $textColor;
font-size: 14px;
}
a {
color: $linkColor;
b {
text-decoration: underline;
}
&:hover {
color: saturate($linkColor, 1.2);
color: desaturate($linkColor, 1.2);
color: darken($linkColor, 1.2);
color: lighten($linkColor, 1.2);
color: opacify($linkColor, 0.5);
}
}
Mixins:
@mixin theme($theme: black) {
background: $theme;
color: #fff;
}
.info {
@include theme;
}
.alert {
@include theme($theme: red);
}
Git / GitHub
For a more detailed explanation check out my git tutorial.
New Project:
git init initalizes your current folder as a git repository git remote add origin <https link> connects your local folder with the GitHub repository you have created on github.com git add . stages all your files git commit -m "First Commit" commits the staged files git branch -M main renames the branch to 'main' git push --set-upstream origin main pushes (= uploads) your local files to your GitHub repository git pull --set-upstream origin main pulls (= downloads) the files in your GitHub repository into your local folder
Staging, Committing, Pushing:
git status shows the status of every changed file git add <file> stages a file git add . stages all changed files git commit -m "Example message" commits the staged files git commit --amend -m "an updated commit message" adds the staged files to the previous commit git commit --amend --no-edit adds the staged files to the previous commit, without changing the commit message git push pushes (= uploads) your local files to your GitHub repository
Undoing:
git revert --no-edit <commit_hash> revert a commit as if done by hand git reset <previous_commit_hash> revert a commit as if it never happened git restore --staged <file> unstages a file git reset HEAD -- <file> same as above (unstages a file) git restore <file> discards changes in an unstaged file git restore . discards all changes in all unstaged files
Branches:
git branch shows branches git switch -c <new-branch> makes a new branch and checks it out (currently changed files will be kept) git checkout -b <new-branch> same as above (old version of command)
Misc.:
git pull pulls (= downloads) the files in your GitHub repository into your local folder git log show a log of all commits git log --oneline show a log of all commits (shorter) git diff shows all changes git diff <a> shows all changes in a file/folder git -v shows the git version you are using git remote -v shows all remotes git remote remove <name> deletes a remote
npm
For a more detailed explanation check out my npm tutorial.
Installing Packages:
npm install <package> installs a package as a dependency npm install --save-dev <package> installs a package as a dev dependency npm uninstall <package> uninstalls a package npm install installs all dependencies npm update installs and updates all dependencies
Misc.:
npm run <custom command name> runs custom command defined in package.json node -v shows node version npm -v shows npm version npm init created package.json for you npm cache clean --force clears cache npm list -g --depth=0 shows all installed packages npmoutdated shows all outdated packages npm view <package> version shows the newest available version of a package
Example package.json
:
{
"name": "project-name",
"private": true,
"devDependencies": {
},
"scripts": {
"build": "webpack",
"watch": "webpack --watch"
}
}
Versions:
1.2.3
: only version 1.2.3, nothing else^1.2.3
: version 1.2.3 or higher, but below 2.0~1.2.3
: version 1.2.3 or higher, but below 1.31.x.x
: version 1.0.0 or higher, but below 2.01.2.x
: version 1.2.0 or higher, but below 1.3*
: any version
Webpack
For a more detailed explanation check out my webpack tutorial.
Installation:
npm install --save-dev webpack webpack-cli installs webpack npm install --save-dev style-loader css-loader sass-loader sass mini-css-extract-plugin installs packages required for (s)css handling npm list --depth=0 | findstr webpack shows webpack version
Running:
npx webpack builds your files npx webpack --watch watches your files (and builds them when a change happens) npx webpack --config webpack2.config.js runs webpack using a specific configuration file
Example webpack.config.js
:
const path = require("path");
const MiniCssExtractPlugin = require("mini-css-extract-plugin");
module.exports = {
// This enables production mode, which minifies the JS and CSS files for fast loading times:
mode: "production",
// Define entry file (which loads every other file):
entry: "./src/index.js",
// Define output file/directory:
output: {
filename: "main.js",
path: path.resolve(__dirname, "dist"),
assetModuleFilename: "assets/[path][name][ext]", // Preserve original file paths and file names for resources (images, fonts, etc.)
},
module: {
rules: [
// Rule for .css files:
{
test: /\.css$/i,
use: [
MiniCssExtractPlugin.loader, // extracts css into a file
"css-loader", // necessary to load css
],
},
// Rule for .scss files:
{
test: /\.scss$/i,
use: [
MiniCssExtractPlugin.loader, // extracts css into a file
"css-loader", // necessary to load css
"sass-loader", // turns scss into css
],
},
],
},
plugins: [
// define name for generated css files:
new MiniCssExtractPlugin({
filename: "[name].css",
}),
],
// Generate source maps for easier debugging:
devtool: "source-map",
};
(Replace MiniCssExtractPlugin.loader
with "style-loader"
to inject CSS into DOM.)
SCSS:
@import "css/example";
JavaScript:
import "./main.scss";
import { doExample } from "./js/example.js";
document.addEventListener("DOMContentLoaded", () => {
doExample();
});
export function doExample() {
console.log("This example works too!");
}
11ty (Eleventy)
For a more detailed explanation check out my eleventy tutorial.
Installation:
npm install --save-dev @11ty/eleventy installs eleventy
Running:
npx @11ty/eleventy builds your files npx @11ty/eleventy --watch watches your files (and builds them when a change happens) npx @11ty/eleventy --serve watches your files (and builds them when a change happens), and stars a webserver on localhost
Example .eleventy.js
:
module.exports = function (eleventyConfig) {
// This makes the eleventy command quieter (with less detail)
eleventyConfig.setQuietMode(true);
// This will stop the default behaviour of foo.html being turned into foo/index.html
eleventyConfig.addGlobalData("permalink", "{{ page.filePathStem }}.html");
// This will make Eleventy not use your .gitignore file to ignore files
eleventyConfig.setUseGitIgnore(false);
// This will copy this folder to the output without modifying it at all
eleventyConfig.addPassthroughCopy("content/assets");
// This defines which files will be copied
eleventyConfig.setTemplateFormats(["html", "njk", "txt", "js", "css", "xml", "json"]);
// This defines the input and output directories
return {
dir: {
input: "content",
output: "public",
},
};
};
Nunjucks:
Setting a variable:
{% set myText = "Hello World!" %}
{% set showHeader = true %}
Outputting a variable:
{{ myText }}
Outputting a variable (without escaping HTML):
{{ myHTML | safe }}
If:
{% if showHeader %}
<header>...</header>
{% endif %}
If/Else:
{% if hungry %}
I am hungry
{% elseif tired %}
I am tired
{% else %}
I am good!
{% endif %}
For Loop:
{% for item in items %}
<li>{{ item.title }}</li>
{% else %}
<li>This would display if the 'item' collection were empty</li>
{% endfor %}
Include:
{% include 'menu.njk' %}
Logical Operators (and, or, not instead of && || !)
{% if myVariable1 and myVariable2 %}...{% endif %}
{% if myVariable1 or myVariable2 %}...{% endif %}
{% if not myVariable %}...{% endif %}
Example Template (e.g. _includes/myTemplate.njk
):
<!DOCTYPE html>
<html lang="en">
<head>
<title>{{ title }}</title>
<script src="{{ nesting }}main.js"></script>
<meta content="text/html;charset=utf-8" http-equiv="Content-Type" />
<meta content="utf-8" http-equiv="encoding" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link href="{{ nesting }}favicon.ico" rel="icon" type="image/x-icon" />
</head>
<body class="{{ bodyClass }}">
<header>Header</header>
<main>
{{ content | safe }}
</main>
<footer>Footer</footer>
</body>
</html>
Example page.html
or page.njk
:
---
layout: myTemplate.njk
title: About Me
bodyClass: about-me-page
nesting: "../"
---
<h1>About Me</h1>
<p>Here is the main content of your file...</p>
I spend hours of my free time writing pages like these that I publish for free. If you'd like to say thanks, please share this tutorial with others and/or buy me a coffee!
If you have questions about this page feel free to contact me via my guestbook or my neocities profile. I promise I don't bite!
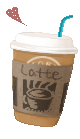